In this article, I covered K6 testing and provided a step-by-step guide on how to integrate it with Playwright. In modern testing scenarios, we will understand how to extract performance metrics using Playwright.
When it comes to Java + Selenium, we can use JMeter to easily integrate performance testing into a project. However, when working with Playwright or Cypress, performance testing requires a different approach. Since these tools primarily focus on functional and end-to-end testing, we need to integrate K6, a powerful open-source tool designed for performance testing, to measure API response times, load handling, and system stability.
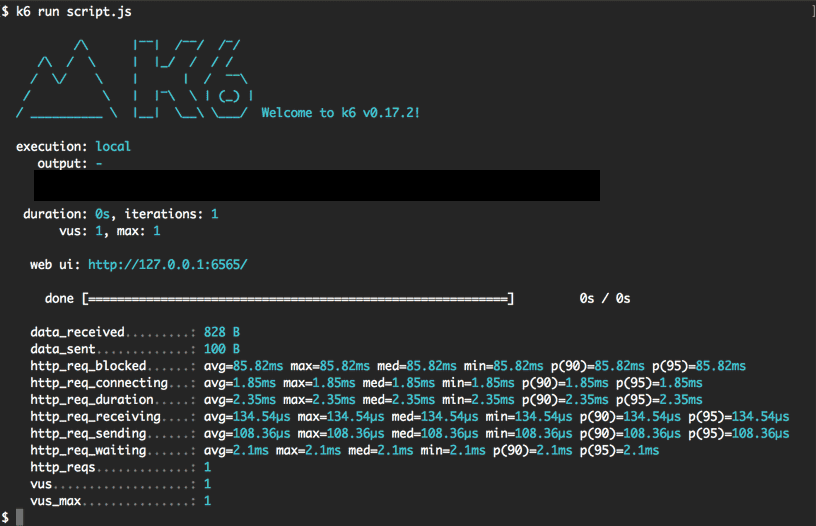
Introduction
Performance testing plays a crucial role in software testing, ensuring that APIs and web applications can handle high loads efficiently. If your application has APIs serving thousands of requests, it’s important to verify their response times and stability under heavy traffic.
Playwright is a popular tool for functional and end-to-end testing, widely used for testing web applications. However, it lacks performance testing capabilities. This is where K6, an open-source performance testing tool, comes in.
What is K6?
K6 is an open-source performance testing tool designed to assess system reliability, scalability, and response times under different load conditions. Developed by Grafana Labs, K6 is widely used for load testing APIs, web applications, and microservices.
Unlike traditional performance testing tools like JMeter, K6 is developer-friendly and uses JavaScript, making it an excellent choice for modern automation frameworks like Playwright and Cypress.
- https://grafana.com/docs/k6/latest
- https://isitobservable.io/site-reliability-engineering/how-to-get-started-with-k6#:~:text=k6%20is%20an%20open%20source,Site%20Reliability%20Engineering
Key Features of K6:
- Scriptable in Typescript – Easily integrates with frontend testing tools.
- Lightweight and CLI-based – Runs tests efficiently without a GUI.
- Scalable Load Testing – Simulates thousands of concurrent users.
- Detailed Performance Metrics – Provides insights on response times, and system behavior.
- CI/CD Integration – Supports Jenkins, GitHub Actions, and GitLab CI/CD.
Why Use K6 with Playwright?
Playwright is primarily a functional and UI testing tool, meaning it lacks built-in performance testing capabilities. By integrating K6 with Playwright, we can extract critical performance metrics, ensuring that APIs and UI interactions meet expected performance benchmarks.
Feature | Playwright | K6 | Why Use Both Together? |
---|---|---|---|
Functional Testing | Playwright tests UI workflows. | ||
Performance Testing | K6 provides load testing for APIs & UI. | ||
API Testing | Both can test APIs, but K6 focuses on load testing. | ||
Real-time Performance Metrics | K6 tracks response times, latency, and throughput. | ||
CI/CD Integration | Both support | ||
JavaScript-based | Easy to integrate for testing. | ||
Simulating Users | K6 helps test high user loads. |
Note: Since Playwright is used for functional and UI automation testing, it lacks built-in performance testing features. By integrating K6, we can bridge this gap and achieve performance testing.
Setting Up Playwright and K6
Before running performance tests, we need to install K6 in our Playwright automation framework.
Prerequisites
Node.js (LTS version)
Playwright (if not installed, run
npx playwright install
)K6 CLI
Step 1: Install Playwright
If you haven’t installed Playwright, initialize it using:
npm init playwright@latest
Or manually install it:
npm install @playwright/test
Step 2: Install K6
K6 is a standalone tool. Install it based on your OS:
brew install k6 // Mac
choco install k6 // Windows
sudo apt update && sudo apt install k6 // Linux
Verify installation:
k6 version // terminal or CMD to type and check it
3. Writing Playwright API Tests
First, let’s write a Playwright test to check an API response.
- Step 1: Create
api.spec.t
s
Create a new file api.spec.ts
in your Playwright project and add the following test :
import { test, expect } from '@playwright/test';
test('Validate API response', async ({ request }) => {
const response = await request.get('https://jsonplaceholder.typicode.com/posts/1');
expect(response.status()).toBe(200);
const data = await response.json();
console.log(data);
});
- Step 2: Run the Playwright API Test
npx playwright test api.spec.ts
If the API is working correctly, it should return status 200 and display JSON data.
4. Generating API Request Data for K6
Now, let’s store the API request details dynamically for K6.
- Step 1: Modify
api.spec.ts
to Save API Details
Update your api.spec.ts
file:
import { test, expect } from '@playwright/test';
import fs from 'fs';
test('Save API request details', async ({ request }) => {
const response = await request.get('https://jsonplaceholder.typicode.com/posts/1');
const requestData = {
url: 'https://jsonplaceholder.typicode.com/posts/1',
method: 'GET',
};
fs.writeFileSync('k6/apiRequest.json', JSON.stringify(requestData, null, 2));
});
- Step 2: Run the Test Again
npx playwright test api.spec.ts
This will generate a new file, apiRequest.json
, containing API request details.
Writing the K6 Performance Test
Now, we’ll use K6 to test the API under load.
Step 1: Create k6-test.js
Create a new file k6-test.js
in your project and add:
import http from 'k6/http';
import { check, sleep } from 'k6';
import { readFileSync } from 'fs';
// Read API request details from JSON file
const apiRequest = JSON.parse(readFileSync('apiRequest.json'));
export let options = {
vus: 10, // 10 Virtual Users
duration: '30s', // Run test for 30 seconds
};
export default function () {
let res = http.request(apiRequest.method, apiRequest.url);
check(res, {
'Status is 200': (r) => r.status === 200,
});
sleep(1);
}
Step 2: Run the K6 Performance Test:
k6 run k6-test.js
Analyzing K6 Test Results
Once the test completes, K6 will display results like:
checks.......................: 100.00% ✓ 200 ✗ 0
http_req_duration...........: avg=200ms min=150ms max=250ms
vus..........................: 10
http_reqs....................: 1000 requests made in 30s
Automating Playwright + K6 in CI/CD
To run these tests in basic CI/CD, use GitHub Actions.
GitHub Actions Workflow
(.github/workflows/ci-cd.yml)
name: API Test and Performance Test
on: [push]
jobs:
test:
runs-on: ubuntu-latest
steps:
- name: Checkout Repository
uses: actions/checkout@v3
- name: Install Node.js
uses: actions/setup-node@v3
with:
node-version: 16
- name: Install Dependencies
run: npm install
- name: Run Playwright API Test
run: npx playwright test api.spec.ts
- name: Install K6
run: |
sudo apt update
sudo apt install k6
- name: Run K6 Performance Test
run: k6 run k6-test.js
As a Fresher QA Engineer, learning API testing with Playwright and performance testing with K6 will give you a strong foundation in automation and performance testing.
If you want, I can guide you step by step on how to improve your API automation skills and how to write efficient performance tests with K6.